Access Modifiers for Class Members
By specifying member access modifiers, a class can control which information is accessible to clients (i.e., other classes). These modifiers help a class define a contract so that clients know exactly which services are offered by the class.
The accessibility of a member in a class can be any one of the following:
- public
- protected
- package access (also known as package-private and default access), when no access modifier is specified
- private
In the following discussion of access modifiers for members of a class, keep in mind that the member access modifier has meaning only if the class (or one of its subclasses) is accessible to the client. Also, note that only one access modifier can be specified for a member.
The discussion in this subsection applies to both instance and static members of top-level classes.
In UML notation, when applied to member names the prefixes +, #, and – indicate public, protected, and private member access, respectively. No access modifier indicates package access for class members.
The package hierarchy shown in Figure 6.5 is implemented by the code in Example 6.8. The class Superclass1 at (1) in pkg1 has two subclasses: Subclass1 at (3) in pkg1 and Subclass2 at (5) in pkg2. The class Superclass1 in pkg1 is used by the other classes (designated as Client 1 to Client 4) in Figure 6.5.
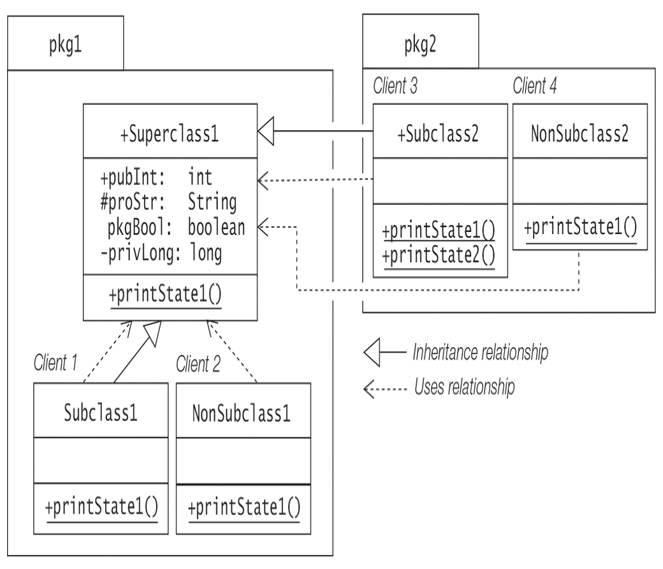
Figure 6.5 Accessibility of Class Members
Accessibility of a member is illustrated in Example 6.8 by the four instance fields defined in the class Superclass1 in pkg1, where each instance field has a different accessibility. These four instance fields are accessed in a Superclass1 object created in the static method printState1() declared in five different contexts:
- Defining class: The class in which the member is declared—that is, pkg1.Superclass1 in which the four instance fields being accessed are declared
- Client 1: From a subclass in the same package—that is, pkg1.Subclass1
- Client 2: From a non-subclass in the same package—that is, pkg1.NonSubclass1
- Client 3: From a subclass in another package—that is, pkg2.Subclass2
- Client 4: From a non-subclass in another package—that is, pkg2.NonSubclass2
Example 6.8 Accessibility of Class Members
// File: Superclass1.java
package pkg1;
import static java.lang.System.out;
public class Superclass1 { // (1)
// Instance fields with different accessibility:
public int pubInt = 2017;
protected String proStr = “SuperDude”;
boolean pgkBool = true;
private long privLong = 0x7777;
public static void printState1() { // (2)
Superclass1 obj1 = new Superclass1();
out.println(obj1.pubInt);
out.println(obj1.proStr);
out.println(obj1.pgkBool);
out.println(obj1.privLong);
}
}
// Client 1
class Subclass1 extends Superclass1 { // (3)
public static void printState1() {
Superclass1 obj1 = new Superclass1();
out.println(obj1.pubInt);
out.println(obj1.proStr);
out.println(obj1.pgkBool);
out.println(obj1.privLong); // Compile-time error! Private access.
}
}
// Client 2
class NonSubclass1 { // (4)
public static void printState1() {
Superclass1 obj1 = new Superclass1();
out.println(obj1.pubInt);
out.println(obj1.proStr);
out.println(obj1.pgkBool);
out.println(obj1.privLong); // Compile-time error! Private access.
}
}
// File: Subclass2.java
package pkg2;
import pkg1.Superclass1;
import static java.lang.System.out;
// Client 3
public class Subclass2 extends Superclass1 { // (5)
public static void printState1() { // (6)
Superclass1 obj1 = new Superclass1(); // Object of Superclass1
out.println(obj1.pubInt);
out.println(obj1.proStr); // (7) Compile-time error! Protected access.
out.println(obj1.pgkBool); // Compile-time error! Package access.
out.println(obj1.privLong); // Compile-time error! Private access.
}
public static void printState2() { // (8)
Subclass2 obj2 = new Subclass2(); // (9) Object of Subclass2
out.println(obj2.pubInt);
out.println(obj2.proStr); // (10) OK! Protected access.
out.println(obj2.pgkBool); // Compile-time error! Package access.
out.println(obj2.privLong); // Compile-time error! Private access.
}
}
// Client 4
class NonSubclass2 { // (11)
public static void printState1() {
Superclass1 obj1 = new Superclass1();
out.println(obj1.pubInt);
out.println(obj1.proStr); // Compile-time error! Protected access.
out.println(obj1.pgkBool); // Compile-time error! Package access.
out.println(obj1.privLong); // Compile-time error! Private access.
}
}